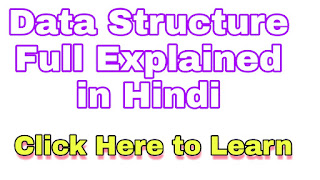
Data Structure - Full Explaination In Detail With Example - Learnengineeringforu
Data Structure Full Explaination
Lets learn data structure with this article, so lets start with array.
Following are the basic topic which we cover in this article.
- ANALYSIS OF ALGORITHMS | PART 2 (WORST, AVERAGE AND BEST CASES
- ANALYSIS OF ALGORITHMS | PART 3 (ASYMPTOTIC NOTATIONS)
- ANALYSIS OF ALGORITHMS | LITTLE O AND LITTLE OMEGA NOTATIONS
- ANALYSIS OF ALGORITHMS | PART 4 (ANALYSIS OF LOOPS)
- ANALYSIS OF ALGORITHM | SET 4 (SOLVING RECURRENCES)
- ANALYSIS OF ALGORITHM | PART 5 (AMORTIZED ANALYSIS INTRODUCTION)
- What does ‘Space Complexity’ mean?
- ANALYSIS OF ALGORITHMS | SET 1 (ASYMPTOTIC ANALYSIS)
- PSEUDO-POLYNOMIAL ALGORITHMS
- BIT ALGORITHM - LEARNENGINEERINGFORU
- PATTERN SEARCHING ALGORITHMS - LEARNENGINEERING
- MATHEMATICAL ALGORITHMS - FULL EXPLANATION - LEARNENGINEERINGFORU
- RANDOMIZED ALGORITHMS IN COMPUTER SCIENCE - FULL EXPLANATION - LEARNENGINEERINGFORU
- GEOMETRIC ALGORITHM IN COMPUTER SCIENCE - LEARNENGINEERINGFORU
- DATA STRUCTURE DYNAMIC ALGORITHM WITH EXAMPLES - LEARNENGINEERINGFORU
- DATA STRUCTURE DIVIDE AND CONQUER WITH EXAMPLES - LEARNENGINEERINGFORU
- INTRODUCTION TO BACKTRACKING ALGORITHM - LEARNENGINEERINGFORU
- BRANCH AND BOUND ALGORITHM EXPLAINATION - LEARNENGINEERINGFORU
- EXPLAINATION OF GREDDY ALGORITHM WITH EXAMPLE - LEARNENGINEERINGFORU
- C PROGRAMMING ARRAY - EXPLAINATION IN DETAIL - LEARNENGINEERINGFORU
- SINGLY LINKED LISTS - DATA STRUCTURE - LEARNENGINEERINGFORU
- DOUBLY LINKED LISTS - DATA STRUCTURE - LEARNENGINEERINGFORU
- CIRCULAR LINKED LISTS - DATA STRUCTURE - LEARNENGINEERINGFORU
- DATA STRUCTURE LINKED LISTS - SINGLY,DOUBLY AND CIRCULAR LINKED LISTS -LEARNENGINEERINGFORU
- TYPES OF ARRAY IN DATA STRUCTURE - LEARNENGINEERINGFORU
- DECLARATION OF ARRAY IN DATA STRUCTURE - LEARNENGINEERINGFORU
- DIFFERENT METHODS OF INITIALIZING 1-D ARRAY - LEARNENGINEERINGFORU
- MULTIDIMENSIONAL ARRAY IN DATA STRUCTURE - LEARNENGINEERINGFORU
- 2D ARRAY INITIALIZATION IN DATA STRUCTURE - LEARNENGINEERINGFORU
- DATA STRUCTURE AND ALGORITHM STACK - LEARNENGINEERINGFORU
- QUEUE PRAGRAM IN C LANGUAGE - LEARNENGINEERINGFORU
- BINARY TREES IN DATA STRUCTURE | ALGORITHM | LEARNENGINEERINGFORU
- DATA STRUCTURE AND ALGORITHM BINARY SEARCH TREES | LEARNENGINEERINGFORU
- DATA STRUCTURE AND ALGORITHM HEAP TREE - LEARNENGINEERINGFORU
- DATA STRUCTURE AND ALGORITHM HASHING - LEARNENGINEERINGFORU
- DATA STRUCTURE - GRAPH DATA STRUCTURE - LEARNENGINEERINGFORU
- DATA STRUCTURE - WHAT IS A MATRIX? EXPLAIN ITS USES WITH AN EXAMPLE - LEARNENGINEERINGFORU
- STRING DATA STRUCTURE - LEARNENGINEERINGFORU